We can create a slideshow using HTML and CSS by following the steps below:
first we need to create the Html structure
Creating the HTML structure
Create the HTML structure for the slideshow. This typically involves creating a container element which will hold the slideshow and individual slide elements.
Html
<div class="slideshow">
<div class="slide">
<h1>Hi, i am content number 1</h1>
</div>
<div class="slide">
<h1>Hi, i am content number 2</h1>
</div>
<div class="slide">
<h1>Hi, i am content number 3</h1>
</div>
</div>>
Apply CSS styles to create the desired appearance and functionality for the slideshow.
Css
.slideshow {
position: relative;
overflow: hidden;
width: 80%;
height: 300px; /* Adjust height as needed */
margin: 0 auto;
}
.slide {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 0.5s ease-in-out;
text-align: center;
} /* Add styles for each individual slide */
.slide:nth-child(1) {
background-color: purple;
}
.slide:nth-child(2) {
background-color: pink;
}
.slide:nth-child(3) {
background-color: gray;
} /* Apply styles to control the slideshow animation */
.slide.active {
opacity: 1;
}
Add JavaScript for slideshow functionality (optional)
If you want to add automatic transitioning between slides or navigation controls, you can use JavaScript.
Here’s an example using JavaScript to add a basic slideshow functionality:
JavaScript
var slides = document.querySelectorAll('.slide');
var currentSlide = 0;
function showSlide(index) {
slides[currentSlide].classList.remove('active');
slides[index].classList.add('active');
currentSlide = index;
}
setInterval(function () {
var nextSlide = (currentSlide + 1) % slides.length;
showSlide(nextSlide);
}, 3000);
Result
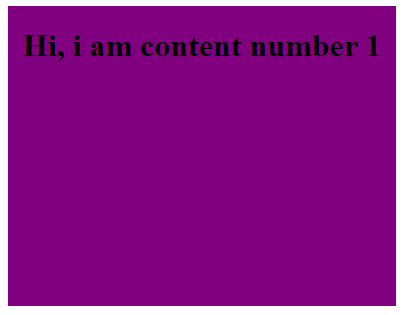
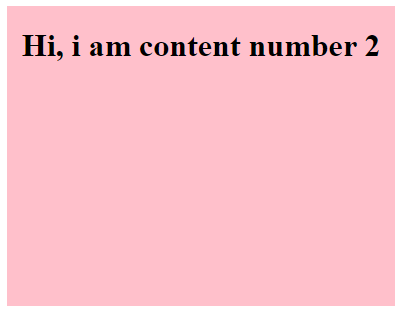
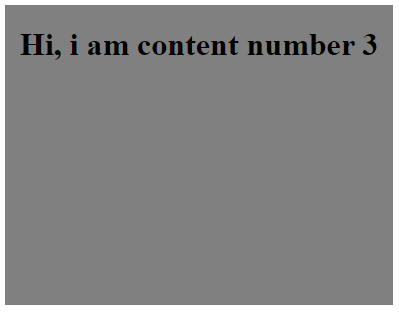
// You can modify the time interval (in milliseconds) between slide transitions.
You can learn more about slideshow here
This is just a simple way to create a slideshow using Html and Css. You can also learn how to create a fade animation in Css
In this example, the slideshow automatically transitions to the next slide every 3 seconds. You can modify the CSS styles and JavaScript functionality to suit your specific design and requirements.
Remember to adjust the content, styles, and JavaScript as per your desired slideshow design and functionality.